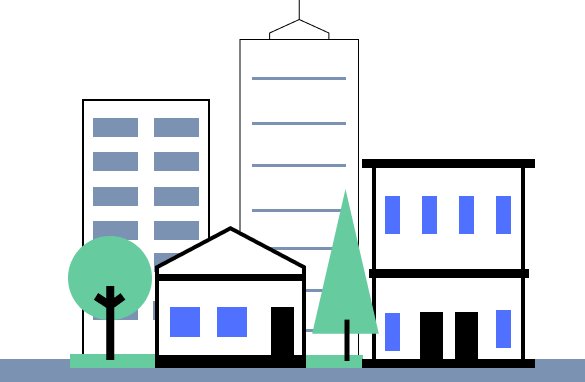
Intro to Exploring LA's Building Permit Data
Recently, I began listening to the podcast There Goes the Neighborhood and its second season explores Los Angeles’ development boom, affordable housing crisis, and gentrification. One way to track and map all this development is thanks to the city’s open data portal which allows users to search, view, download, and problematically access data via Socrata’s API. In this post, we will explore the city’s building permit dataset, its schema, and accessing it from the API.
We will be working the the Building Permits dataset and start exploring it through the API. For documentation on constructing url queries refer to Socrata’s SODA API. The base url we will be using is https://data.lacity.org/resource/nbyu-2ha9.json and we will work with downloading the dataset/subsets and transform the response into geojson. Future posts will start exploring opportunities on how to create/serve map tiles from the data, producing layers from analysis, and creating an automated update cycle.
For this example we will be using Node.js to dowload and filter the data so take a look at their documentation on installing node.
Use node.js to download the data
var fs = require('fs');
var needle = require('needle');
var baseUrl = 'https://data.lacity.org/resource/nbyu-2ha9.json';
var query = "?$where=issue_date='2017-10-19T00:00:00'";
var outputFile = '/tmp/permits.json'
// Function to download and write data to json file
function getData(url, output callback) {
needle.get(url, function(error, response) {
if (error) return callback(error);
var dataString = JSON.stringify(response.body, null, 2);
fs.writeFile(output, dataString, function(error) {
if (error) return callback(error);
return callback(null, 'Finished writing file to ' + output);
});
});
}
// Run function to download data where issue data is October 19, 2017
var urlQuery = baseUrl + query;
getData(urlQuery, outputFile, function(error, respose) {
if (error) return console.error(error);
return console.log(response);
});
// Finished writing file to /tmp/permits.json
// The output file json will be an array of 1000 permits
// Default request will be 1000 records
This is the initial exploration of downloading permit data. Next we will continue to explore, map, and analyze the dataset for insight into Los Angeles’ development. Here’s the dataset subset mapped thanks to geojson.io and bl.ocks.org